Content
Overview
A Checker
has a set of Filter
s that decide which audit events
the Checker
reports through its listeners. Interface Filter
and class FilterSet
are intended to support general AuditEvent
filtering using a set of Filter
s.
A Filter
has boolean
method accept(AuditEvent)
that
returns true
if the Filter
accepts the AuditEvent
parameter and returns false
if the Filter
rejects it.
A FilterSet
is a particular Filter
that contains a set of
Filter
s. A FilterSet
accepts an AuditEvent
if and
only if all Filter
s in the set accept the AuditEvent
.
Here is a UML diagram for interface Filter
and class FilterSet
.
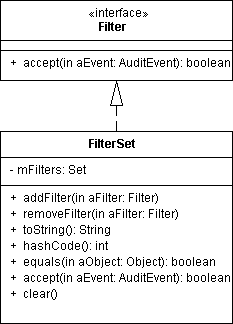
Writing Filters
The Filter
that we demonstrate here rejects
audit events for files whose name matches a Pattern. In order to
enable the specification of the file name pattern as a property in a
configuration file, the Filter
is an AutomaticBean
with mutator method setFiles(String)
that
receives the file name pattern. An AutomaticBean
uses JavaBean
introspection to set JavaBean properties such as files
.
package com.mycompany.filters;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
import com.puppycrawl.tools.checkstyle.api.AuditEvent;
import com.puppycrawl.tools.checkstyle.api.AutomaticBean;
import com.puppycrawl.tools.checkstyle.api.Filter;
import com.puppycrawl.tools.checkstyle.api.Utils;
public class FilesFilter
extends AutomaticBean
implements Filter
{
private Pattern mFileRegexp;
public FilesFilter()
throws PatternSyntaxException
{
setFiles("^$");
}
public boolean accept(AuditEvent aEvent)
{
final String fileName = aEvent.getFileName();
return ((fileName == null) || !mFileRegexp.matcher(fileName).find());
}
public void setFiles(String aFilesPattern)
throws PatternSyntaxException
{
mFileRegexp = Utils.getPattern(aFilesPattern);
}
}
Declare check's external resource locations
Using Filters
To incorporate a Filter
in the filter set
for a Checker
, include a module element for
the Filter
in the configuration file. For example, to
configure a Checker
so that it uses custom
filter FilesFilter
to prevent reporting of
audit events for files whose name contains "Generated",
include the following module in the configuration file:
<module name="com.mycompany.filters.FilesFilter">
<property name="files" value="Generated"/>
</module>
Huh? I can't figure it out!
That's probably our fault, and it means that we have to provide better documentation. Please do not hesitate to ask questions on the user mailing list, this will help us to improve this document. Please ask your questions as precisely as possible. We will not be able to answer questions like "I want to write a filter but I don't know how, can you help me?". Tell us what you are trying to do (the purpose of the filter), what you have understood so far, and what exactly you are getting stuck on.
Contributing
We need your help to keep improving Checkstyle. Whenever you write a filter that you think is generally useful, please consider contributing it to the Checkstyle community and submit it for inclusion in the next release of Checkstyle.